How do I manage local users and groups?
Table of Contents
I. Introduction
In this chapter, we'll learn how to manage Windows local users and groups using PowerShell! A native PowerShell module named "Microsoft.PowerShell.LocalAccounts" is used to create, modify and delete local users and groups in the Windows local account database (SAM database).
The "Microsoft.PowerShell.LocalAccounts" module, which we can call "LocalAccounts", has been available on Windows and Windows Server since the release of Windows 10 and Windows Server 2016, so you should have it on the vast majority of your machines. In a way, this module is the successor (or competitor...) of the famous "net user" and "net localgroup" commands. By the way, these commands still work.
II. LocalAccounts module commands
To list the commands of the LocalAccounts module and get an idea of its potential, we will use this command:
Get-Command -Module Microsoft.PowerShell.LocalAccounts
This module contains a dozen commands, listed below:
- Add-LocalGroupMember: add a user to a local security group
- Disable-LocalUser: disable a local user account
- Enable-LocalUser: activate a local user account
- Get-LocalGroup: obtain a list of local security groups
- Get-LocalGroupMember: obtain a list of security group members
- Get-LocalUser: obtain the list of local users
- New-LocalGroup: create a new security group
- New-LocalUser: create a new local user
- Remove-LocalGroup: remove a local security group
- Remove-LocalGroupMember: remove a member of a local group
- Remove-LocalUser: remove a local user
- Rename-LocalGroup: rename a local group
- Rename-LocalUser: rename a local user
- Set-LocalGroup: modify a local group
- Set-LocalUser: modify a local user, useful for changing the password
You really do have everything you need to manipulate local users and groups!
III. Creating a local user with PowerShell
To begin with, we're going to create a local user with PowerShell. The name of this user will be stored in the "$AccountName" variable, its password in the "$AccountPassword" variable and the description in "$AccountDescription".
The password must meet the requirements of the password policy of the local machine, or of the Active Directory in the case of a domain-integrated machine. Furthermore, if the password is not specified, it must be entered interactively in the PowerShell console.
$AccountName = "test-fb" $AccountPassword = ConvertTo-SecureString "P@ssword123!" AsPlainText -Force $AccountDescription = "Test account" # Create local account New-LocalUser $AccountName -Password $AccountPassword -FullName $AccountName -Description $AccountDescription
The New-LocalUser command will create the "test-fb" account on the local computer. By default, this account is enabled, so if you wish it to be disabled, add the "-Disabled" parameter. Other parameters are available for this command, as mentioned in the Microsoft documentation.
Note: when an account is created with New-LocalUser, it does not belong to any security group. As a result, it will not be possible to open an interactive session on Windows Server (whereas it will be possible on Windows 10 or Windows 11). So, as a complement, you'll need to add it to the Users group at the very least, using the Add-LocalGroupMember command described in the rest of this article.
This new account appears in the list of local accounts:
Get-LocalUser
The proof is in the pictures:
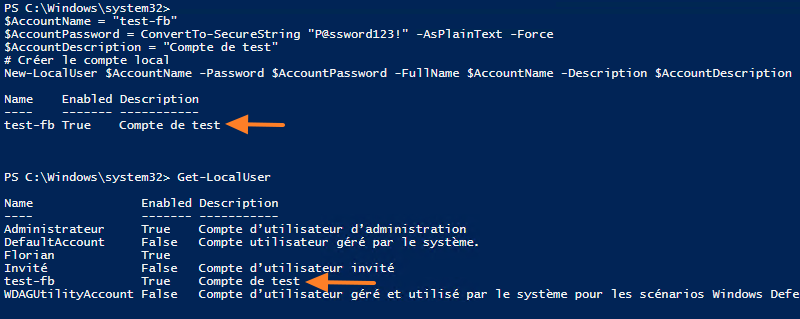
By the way, the "Get-LocalUser" command can return a lot of information about accounts: last login, password expiry date, date of last password change, account SID, etc.... Here's how to get this detail:
To obtain this information for all accounts :
Get-LocalUser | Select-Object *
To obtain this information for an account :
Get-LocalUser -Name"test-fb" | Select-Object *
IV. Disabling the local Administrator account with PowerShell
Let's imagine we want to disable the local "Administrator" account on the Windows machine and use a new account with a customized name. First, we'll add the"test-fb" account to the machine's local "Administrators" security group(yes, I could have chosen a better username).
Using the "Add-LocalGroupMember" cmdlet, we add our user to the "Administrators" group:
Add-LocalGroupMember -Group"Administrators" -Member"test-fb"
Then, if we list the members of the"Administrators" group, we can see that the"test-fb" account is indeed present:
Get-LocalGroupMember -Group"Administrators
Note that on English Windows, the group name will be"Administrators".

All that remains is to deactivate the native "Administrator" account created when the machine was installed:
Disable-LocalUser -Name"Administrator
The "Administrator" account is now disabled on the local machine. Instead, we can use the "test-fb" account! This could be managed by a Windows LAPS strategy! 🙂
V. Managing local groups with PowerShell
First of all, the long list of local security groups built into Windows can be obtained with a simple :
Get-LocalGroup
In the corporate world, we rarely need to create or delete a new local security group. On the other hand, we do regularly need to add or remove members of these groups. For example, if we wish to give administration rights on a machine to a user or group in the Active Directory domain.
To add the account "IT-Connectadmin.fb" (user account on the AD domain) as a member of the "Administrators" group, we will use this syntax:
Add-LocalGroupMember -Group "Administrators" -Member"IT-Connectadmin.fb"
We can also add several elements at once:
Add-LocalGroupMember -Group "Administrators" -Member ("IT-Connectaccount1","IT-Connectaccount2")
The reverse operation, i.e. removing members from this group, is not very complicated: all you have to do is change the name of the command :
Remove-LocalGroupMember-Group "Administrators" -Member ("IT-Connectaccount1","IT-Connectaccount2")
If you need to create a new local security group, use the "New-LocalGroup" command.
VI. Conclusion
Now we've seen some practical commands for managing local users and groups using PowerShell and the Windows built-in module!
In addition to this chapter, you can consult the Microsoft documentation for help on the various commands in this PowerShell module.