The scope of variables
Table of Contents
I. Introduction
In this chapter, we'll look at the scope of PowerShell variables, because in addition to having a type, variables also have a scope, meaning that they can only be accessed in certain parts of your script.
By default, a variable is accessible throughout the script in which it was declared, and even beyond since it is accessible in the entire PowerShell session, but you may not want this for all variables.
II. Different scopes for variables
PowerShell supports 3 different types of scope for its variables: global, local and script. There are also private variables, but these are not really scopes.
Each PowerShell variable is associated with a scope. Here is a summary table:
Variable range | Description |
---|---|
Global | The variable can be accessed anywhere in the PowerShell session, including outside the current script. This is the default scope for variables created in a PowerShell console. It is also associated with automatic variables. |
Local | The variable can only be accessed within the function or script in which it was defined. This is the default scope assigned to a variable in a script (unless a scope name is specified). Once the script has finished executing, the variable is inaccessible. |
Script | The variable can be accessed anywhere in the current script, but not outside it. |
III. Assigning scope to a variable
To assign a scope explicitly to a variable, use the following syntax:
$<Nom de la portée>:<Nom de la variable>
You'll notice that the dollar sign still appears as a prefix, but that the choice of scope via is inserted between this symbol and the variable name.
Which gives:
$Global:VariableGlobale
$Local:VariableLocale
$Script:VariableScript
IV. Examples
To put this notion of scopes into practice with PowerShell variables, we'll take a look at the"Global" and"Script" scopes.
A. Global scope
A variable with "Global" scope is accessible between several scripts. We're going to check this by creating two scripts. A first script where the variable is declared and where we'll display the variable in the console, and a second script which will simply display the contents of the variable.
- Script-1.ps1
$global:Texte = "Hello World"
Write-Host $Texte
- Script-2.ps1
Write-Host $Texte
If we run the first script, then the second, we can see that both scripts have accessed the contents of the variable. This is possible because we're using a variable associated with the"Global" scope.
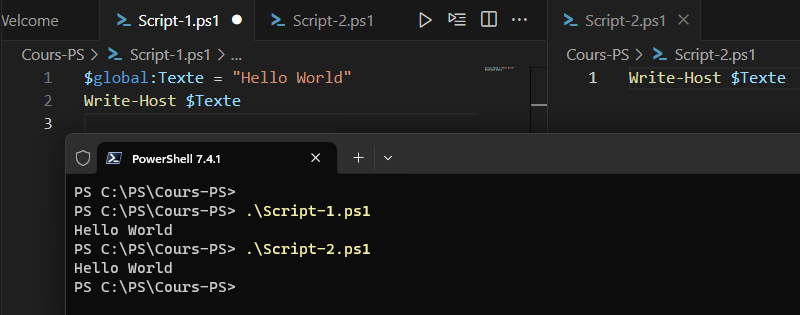
B. Script scope
We're going to carry out the same exercise as with the "Global" scope, but using the "Script" scope (with "Local", the behavior would be identical in this case). So we're going to create two scripts.
- Script-3.ps1
$script:TexteScript = "Hello World"
Write-Host $TexteScript
- Script-4.ps1
Write-Host $TexteScript
We run the first script, where the variable "$TexteScript" is declared, then the second. This time, the "Write-Host" command returns an empty string. This shows that the "$TexteScript" variable is not accessible between scripts.
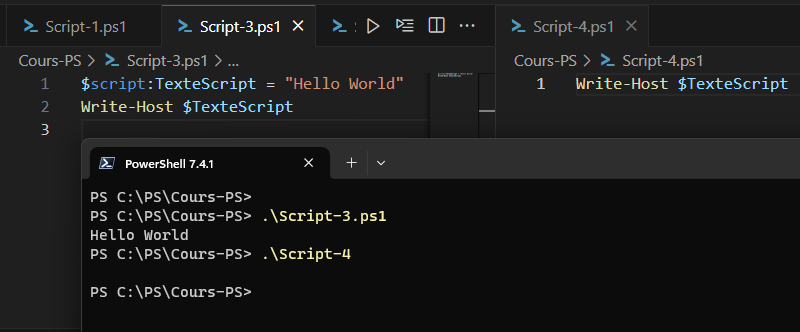
We won't be comparing "Script" and "Local" scopes in this chapter, as we haven't yet covered the notion of PowerShell functions.
V. Conclusion
Although it's not always necessary to play with the scope of PowerShell variables, it is important to be aware of this notion, especially when writing scripts, modules and functions. We often use the "Global" scope to extend the accessibility of a specific variable.