For loops
Table of Contents
I. Introduction
In PowerShell, there are many ways to perform loops, and each type of loop has its own characteristics. In the chapter to follow, we'll focus on PowerShell "For" loops, which are particularly useful for performing an action a set number of times.
The "For" loop is a classic in many scripting and programming languages. This chapter includes a number of examples to help you familiarize yourself with the principle of "For" loops.
II. What is a loop in scripting?
In scripting and programming, a loop is used to repeat a block of code in order to perform identical operations on a set of values. This block of code will be repeated a certain number of times, depending on what we call a repetition condition.
The purpose of a loop is to simplify code by avoiding redundancy. Instead of writing the same code over and over again, you can simply place it in a loop that will execute it as many times as necessary, and do so dynamically (to run through an entire collection, for example).
III. How does a For loop work?
The aim of a "For" loop is to execute a block of instructions X times, where the number of executions depends on the state of the condition being tested.
In PowerShell, the "For" loop is based on the following syntax :
For(<initial state>;<repeat condition>;<increment>) { <If the condition is true, we execute this block of instructions> } <If the condition is false, the for loop ends and the script continues...>
When we use a "For" loop, we follow the following logic: we specify a starting value (initial state), a target value in the repeat condition (for example, the value 10) and we increment the value with each loop turn (each iteration): we can increment by 1, 2, 10, etc., as required.
IV. For" examples in PowerShell
To get the full picture, it's always better to have examples, so let's start with a very simple example. We'll then look at another example with a "dynamic" loop and a third example with two conditions.
- Basic "For" loop
For this first example, we'll assume that we start with the value "1", and as long as the value is less than 10, we'll execute the "For" loop instruction block, incrementing by +1 at each iteration (i.e. each loop turn).
Note: Assigning the value "0..10" to a variable creates an array of values, from 0 to 10.
Which gives:
$value = 0..10 For($i=0 ; $i -lt 10 ; $i++) { Write-Output "The value is $($value[$i])" }
We obtain the following result:
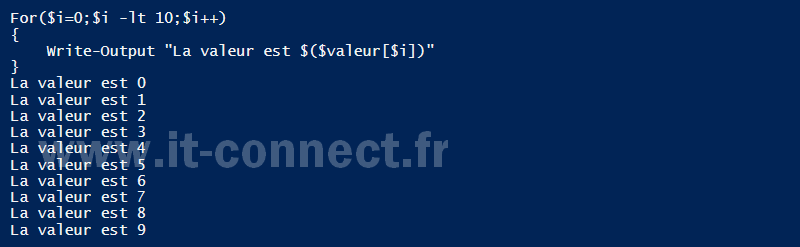
This means that after the line "The value is 9", script execution will continue. The sentence "The value is 10" is not displayed, because we've used the "$i -lt 10" repeat condition, so $i must be strictly less than 10 for the code in the For loop to be executed.
- For" loop based on a number of elements
Let's imagine we have an array of strings and want to execute an action on each value in the array, without knowing in advance the number of elements in the array. We can use the "Length" property to calculate the size of the array (the number of elements in the array).
We're going to define the "$langages" variable and assign values to it (this could be dynamic). Thanks to "$langages.Length", we'll be able to create a loop that processes all the elements in the array. This is what we usually do (and more simply) with a "ForEach" loop, which we'll study in a later chapter, but we can also do it with a simple "Fo "r loop, hence this example.
Which gives:
$langages = @("PowerShell", "Python", "PHP", "JavaScript", "C-Sharp") For($i=0 ; $i -lt $langages.Length ; $i++) { Write-Output "The best programming language is $($langages[$i])" }
We obtain the following result:
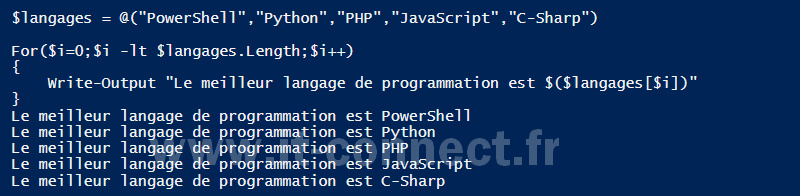
- For" loop with multiple conditions
Within a "For" loop, we generally use the "$i" variable to handle initialization and incrementation (we could use another variable name). If required, we can use two conditions to create a multi-condition "For" loop. For this example, we'll use "$i" and "$j".
To go from one variable to two, and thus have two repeat conditions, we'll need to change the syntax of our "For" loop.
$value = 0..10 For(($i = 0 ), ($j = 0 ); $i -lt 10 -and $j -lt 10 ; $i++ ,$j++ ) { Write-Output "The values are: i = $($value[$i]) - j = $($value[$j])" }
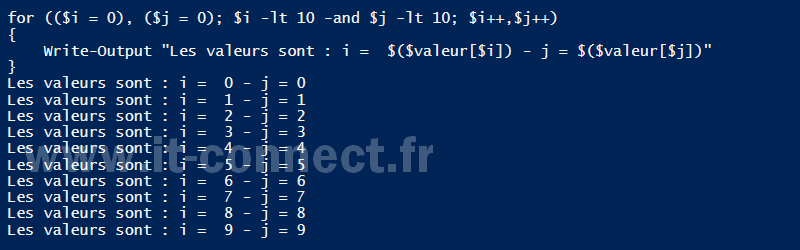
If we take the two previous examples, with the value from 1 to 10 and the language table, we get :
$value = 0..10 $languages = @("PowerShell", "Python", "PHP", "JavaScript", "C-Sharp") For(($i = 0 ), ($j = 0 ); $i -lt 10 -and $j -lt $langages.Length ; $i++ ,$j++ ) { Write-Output "$($value[$i]) - $($langages[$i])" }
As soon as we reach the end of the "$langages" array, we exit the "For" loop, as "$i" must be less than 10, which is always the case AND "$j" must be less than the length of the array, which is no longer the case, as we've reached the end of the array.
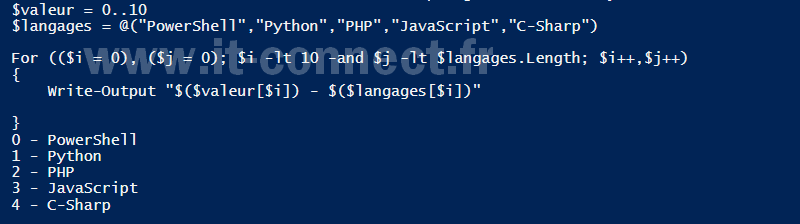
If we want it to continue until the end of "$i" and "$j", we need to replace the "-and" with "-or" (replace "and" with "or") so that it continues as long as either condition is true. The result will then be different:
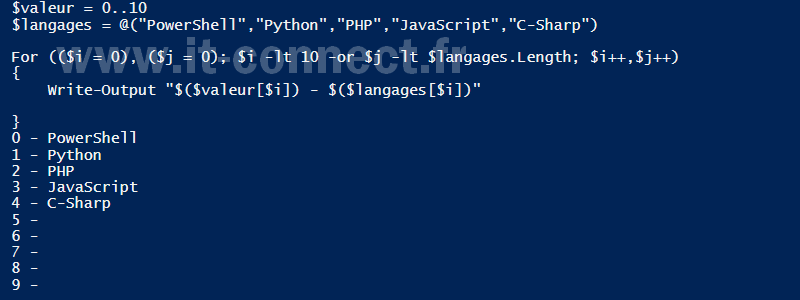
V. Conclusion
For" loops are simple to use and can come in handy in your scripts, but don't forget to use the right type of loop depending on the circumstances: a "Do-While" loop, a "Do-Until" loop or a "ForEach" loop. You'll be better equipped to do this once we've studied the different types of loops, and put them to practical use in PowerShell scripts.