Discovering variables with PowerShell
Table of Contents
I. Introduction
In this chapter, we'll start with the basics, evoking the notion of variable, before moving on to manipulating our first PowerShell variables.
II. What is a variable?
A variable is a container for storing a value. You can think of each variable as a box in which to store an object for easy retrieval at a later date. It can be a string, a number, an array, a complete object, etc... The variables we create are stored in memory and are ephemeral (for the duration of a PowerShell session or script execution).
The advantage is that the variable can be called X number of times in a script, so its value can be consulted each time. In other words, we can access the object stored in the box at any time.
III. Declaring a variable in PowerShell
In PowerShell, declaring a variable is simple and straightforward. You use the dollar sign($) followed by the variable name (which you define), then the equal sign (=), and finally the value you want to store. Here's an example:
$maVariable = "Hello World"
In this example, we've declared a variable called "myVariable" and assigned it the value "Hello World".
Once you've declared a variable, you can use it in your script by specifying its name.
For example:
$maVariable = "Hello World"
Write-Output $maVariable
We could even embed our variable in a complete sentence, with the variable name replaced by its content.
Write-Output "Voici le contenu de ma première variable : $maVariable"
The result is as follows:

So, every time we need to write "Hello World", all we have to do is enter the name "$myVariable": whether it's 1 time, 2 times or 20 times. The advantage is that if we wish to replace the text "Hello World" with "Bonjour le monde", we simply change the value assigned to the variable. Without a variable, we'd have to replace the value as many times as necessary.
Executing the command below will overwrite the contents of the variable. It will no longer be equal to "Hello World" but to "Bonjour le monde".
$maVariable = "Bonjour le monde"
Note: the most direct way to read the contents of a variable is to enter its name in the console and press Enter.
In addition to storing text or a number, a variable can be used to store the result of a command. For example, we can store the list of services in a variable named "$Service" :
$Service = Get-Service
Here's the result in pictures:
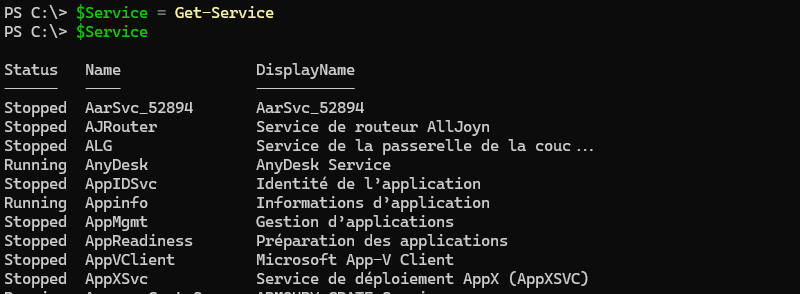
IV. Variable types
Each variable is associated with a type whose purpose is to indicate the format of the data stored in the variable. For example, this can be used to distinguish between text and numbers.
PowerShell supports different types of variable. Here are the main ones:
Variable type | Description |
---|---|
[String] System.String | A character string, i.e. text. Example: $String = "Hello world". |
[Integer] System.Int32 | A whole number. Example: $Number = 7 |
[Double] System.Double | A decimal number (64 bits) Example: $DecimalNumber = 7.5 |
[Boolean] System.Boolean | A Boolean value, i.e. true or false. Example: $Booleen = $true |
[Array] System.Object | An array, which can contain several values. Example 1: $Array = 1,2,3,4 Example 2: $Array = "hello", "good evening", "goodbye". |
[HashTable] System.Object | A hash table, i.e. a collection of key/value pairs. Example: $HashTable = @{"1"="One"; "2"="Two"} |
[DateTime] System.DateTime | A date and/or a time. Example: $Date = Get-Date |
PowerShell is what you might call a dynamically typed language, which means you don't need to specify the data type when declaring a variable. PowerShell will automatically determine the data type based on the value you assign to the variable.
For example, we will define these two variables:
$nombre = 10
$texte = "Bonjour"
Then look up their type using the"GetType()" method. Like this:
$nombre.GetType()
$texte.GetType()
We can see that the variable $number is of type"Int32" while the variable $text is of type"String". This is consistent with the data contained in each variable. We haven't done anything, which proves that PowerShell has performed the typing itself.
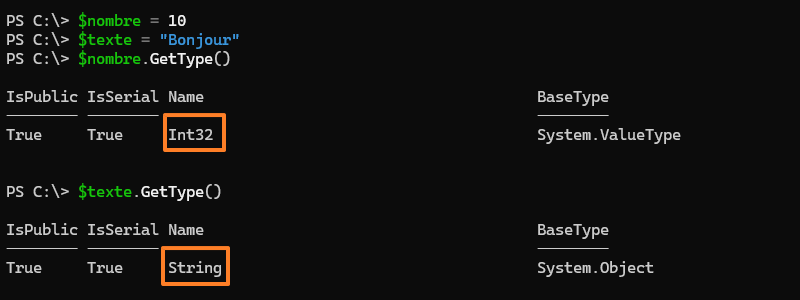
In certain situations, it may be necessary to force the type of a variable. It's possible, and it's very simple. You need to prefix the variable name with the type of your choice. For example, to force the type to "string" :
[string]$nombre = 10
After executing this command, if you look at the variable's type, it will be the expected one (String), whereas under normal circumstances, PowerShell would have assigned the "Int32" type.

Even so, you can't have it both ways: there must be consistency between the variable type and the data to be stored. For example, if you force the type to "[DateTime]", you won't be able to store text such as "Hello", or even a number, as PowerShell expects a date.
V. Concatenate strings
Concatenation is the act of joining two or more strings into a single string. For example, if we have the first name "Florian" on one side and the last name "Burnel" on the other, we could aim to concatenate these two values (i.e. two strings) to form a single string with the full name: Florian Burnel.
There are several ways to concatenate strings. We're going to look at a method based on the "+" operator, as if we were adding values. To return to the example given in the introduction, we'll define two variables with the first and last names. Which gives:
# Prénom
$prenom = "Florian"
# Nom
$nom = "Burnel"
Now, if we want to concatenate these two values to make a single value that will be stored in a new variable named "$lastname", we'll use this syntax:
$prenomnom = $prenom + $nom
The problem is that this syntax will return the following result:
FlorianBurnel
In other words, there's a space missing between the two values! To improve the result, we're going to concatenate these two values with a space that will be positioned between the two strings. Here's the new syntax to obtain this result:
$prenomnom = $prenom + " " + $nom
Since there's a space between the quotation marks, we get a cleaner output:
Florian Burnel
We could imagine the concatenation between a string and our two variables like this:
Write-Host "Je m'appelle $prenom $nom !"
Which gives:
Je m'appelle Florian Burnel !
VI. Conclusion
Now you can declare a variable, use it in your script or in the PowerShell console, and you know that there are several types of variable in PowerShell. Now that you know how to create your own variables, in the next chapter we'll look at automatic variables.