Add controls to function parameters
Table of Contents
I. Introduction
When creating a function in PowerShell, we have the opportunity to add a set of parameters for this function. From now on, we'll learn how to add checks on these function parameters, which will enable us to verify the data sent as input to the function. This will also enable us to make a parameter mandatory or not.
II. Make a parameter mandatory with mandatory
For some of your functions, you may need to make one or more parameters mandatory. Indeed, if you expect a value on a parameter and the user doesn't specify a value, this could "crash" the function and return an unexpected result.
Let's take the "Get-Login" function as an example. This option returns an identifier in the form "<First letter of first name>.<last name>" from a first and last name sent to the function. To do this, we have two parameters: "$Name" and "$Firstname". This identifier must be returned in lower case.
function Get-Login {
param($Nom,
$Prenom)
$Identifiant = (($Prenom).Substring(0,1)).ToLower() + "." + ($Nom).ToLower()
return $Identifiant
}
$Login = Get-Login -Prenom "Florian" -Nom "Burnel"
This PowerShell function returns the expected result: with the first name "Florian" and the last name "Burnel", we obtain the identifier "f.burnel.
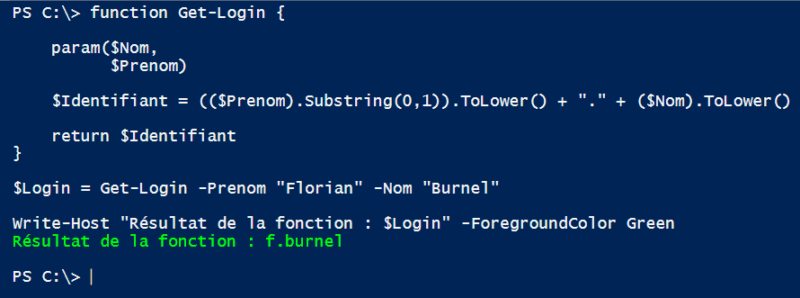
The problem is that, currently, I can call the function omitting one or both values. This will return an error.
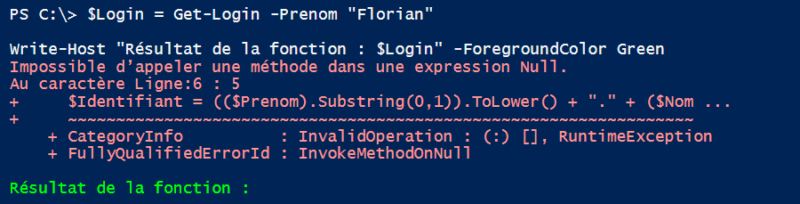
We're going to modify the function to make both parameters mandatory. In this way, the function will refuse to run if the first name and/or surname are missing. We need to add the " [Parameter(Mandatory=$true)]The "Mandatory" parameter is set to "$true". Finally, it's the setting of the "Mandatory" parameter to "$true" that makes it mandatory.
We obtain the following code:
function Get-Login {
param([Parameter(Mandatory=$true)]$Nom,
[Parameter(Mandatory=$true)]$Prenom)
$Identifiant = (($Prenom).Substring(0,1)).ToLower() + "." + ($Nom).ToLower()
return $Identifiant
}
From now on, if we call the function without specifying the first or last name, PowerShell will wait for a value.
$Login = Get-Login -Prenom "Florian"
Here is an example where the function is called without a value for the "-Name" parameter:

If we specify a value and press Enter, the function will be executed. This example illustrates the importance and usefulness of making certain function parameters mandatory.
III. Parameter control
In addition to controlling whether a parameter is mandatory or not, you can add parameter controls. This is very useful to ensure that the value corresponds to a precise format, that the value corresponds to a set of accepted values, that the value respects a certain length, or to execute a command to validate the value.
A. ValidatePattern
To begin with, we'll refine our previous example by adding a check on the "$Lastname" and "$Firstname" parameters to ensure that they contain only letters, in either upper or lower case. This prevents the first name and/or surname from containing a number.
We need to add the "ValidatePattern" parameter, which defines a regular expression to validate the value associated with the parameter in question. Here, we're checking the use of letters, exclusively.
function Get-Login {
param([Parameter(Mandatory=$true)][ValidatePattern("^[a-z]+$")]$Nom,
[Parameter(Mandatory=$true)][ValidatePattern("^[a-z]+$")]$Prenom)
$Identifiant = (($Prenom).Substring(0,1)).ToLower() + "." + ($Nom).ToLower()
return $Identifiant
}
So, if we run this :
$Login = Get-Login -Prenom "Florian1" -Nom "Burnel"
We get an error, because the value "Florian1" doesn't respect the model defined in "ValidatePattern".
Get-Login : Impossible de valider l'argument sur le paramètre «Prenom». L'argument «Florian1» ne correspond pas au modèle «^[a-z]+$». Indiquez un argument qui correspond à «^[a-z]+$ et réessayez.
We could also use "ValidatePattern" to check that the value corresponds to an e-mail address on a specific domain name. For example, the line below ensures that the e-mail address contains "@it-connect.fr". :
param([ValidatePattern("@it-connect.fr$")]$Email)
Note that PowerShell 7 supports the ability to specify a custom message to be returned to the user when validation fails. The syntax is as follows:
[Parameter(Mandatory=$true)][ValidatePattern("^[a-z]+$",ErrorMessage="Valeur incorrecte")]$Nom
In the example above, if the value for the Name parameter is not accepted, PowerShell will return the text "Invalid value".
B. ValidateSet
The "ValidateSet" parameter is used to define a set of accepted values. It is used as follows:
param([ValidateSet(1,2,3,10,20,30,100)]$Nombre)
Within the parentheses, you must indicate the permitted values. If the value of parameter "$Number" is different, then the function will not execute.
C. ValidateLength
The "ValidateLength " parameter is used to define a minimum and maximum length for a parameter. It is used as follows:
param([ValidateSet(1,10)]$Chaine)
In the example above, the value of the "$String" parameter must be between 1 and 10 characters, as the number of characters corresponds to its length.
D. ValidateScript
The "ValidateScript" parameter is used to execute a validation script to accept or reject the value of a parameter. It is used as follows:
param([ValidateScript({Test-Path $_})]$Path)
In the example above, the"$Path" parameter is intended to be the path to a directory. Using the script in"ValidateScript", we'll execute the"Test-Path" command to test the existence of this path: this command will return a Boolean (true or false). If the path is valid, the value will be accepted and the function will run!
E. ValidateNotNullOrEmpty
The "ValidateNotNullOrEmpty" parameter is used to indicate that this parameter will not accept a non-null or empty value. It is used as follows:
param([ValidateNotNullOrEmpty()]$Chaine)
In the example above, the value of the "$Chaine" parameter cannot be empty or null, otherwise we'll get an error. In other words, we can't call the parameter with :
Nom-Fonction -Chaine ""
IV. Conclusion
Thanks to these new concepts, you'll be able to better control and configure your configuration parameters in PowerShell. In the rest of this course, we'll see how you can go even further in configuring parameters!