A cmdlet-like function with CmdletBinding()
Table of Contents
I. Introduction
Simply adding the "[CmdletBinding()]By integrating the "cmdlet" into a function, you give it additional capabilities, like those found in official cmdlets. Let's take a look at how to integrate it into a function and what the expected benefits are.
II. Adding CmdletBinding() to a function
We'll take as our starting point the Get-Login function used in previous chapters. As a reminder, here is the basic code for this function:
function Get-Login {
param($Nom,
$Prenom)
$Identifiant = (($Prenom).Substring(0,1)).ToLower() + "." + ($Nom).ToLower()
return $Identifiant
}
Currently, this function has two parameters, as shown in the image below:
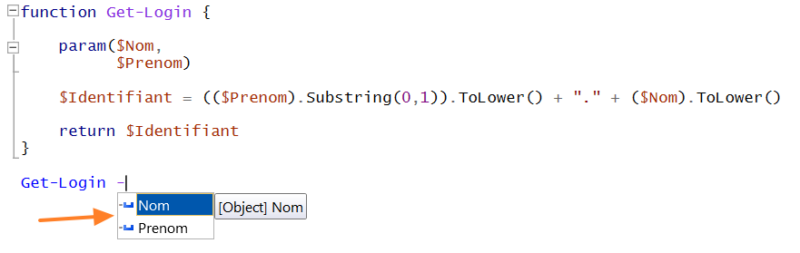
From now on, we'll modify the function code to add the instruction [CmdletBinding()]instruction, as follows:
function Get-Login {
[CmdletBinding()]
param($Nom,
$Prenom)
$Identifiant = (($Prenom).Substring(0,1)).ToLower() + "." + ($Nom).ToLower()
return $Identifiant
}
Then, if we load the function into memory and look at the list of its parameters, we can see a very different result:
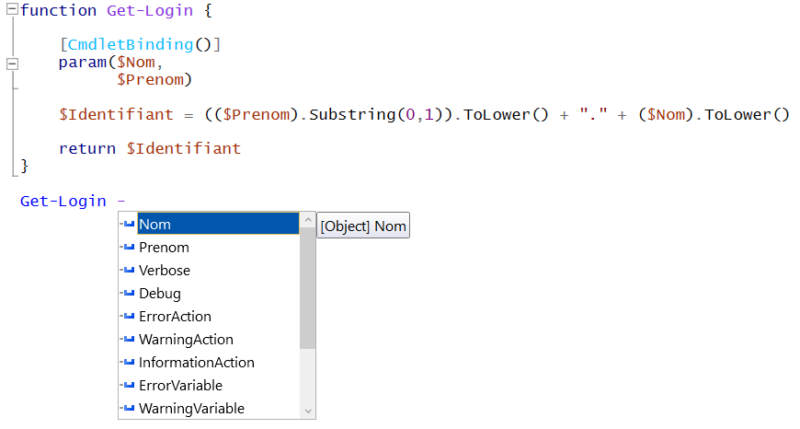
As with PowerShell cmdlets, we can see that our function has various parameters such as ErrorAction, WarningAction, ErrorVariable, Verbose, etc., which can be very useful for handling events and errors in our scripts.
III. Function with Begin, Process, End
Please note that if you wish to send values to a function via the pipeline, you must add the "ValueFromPipeline" instruction to the parameter of your choice, on the same principle as "Mandatory" which we studied earlier.
Here's how to configure a parameter in this way:
param([Parameter(ValueFromPipeline=$true)]$Nombre)
To ensure that all values sent via the pipeline are processed, we also need to revise the function's overall syntax to incorporate three blocks: Begin{}, Process{} and End{}.
A few explanations are in order to explain the benefits of this change:
- Begin{} will be executed only once, on the first function call, before processing the first value.
- Process{} will be executed for each value sent to the function via the pipeline
- End{} will be executed only once, after processing the last value sent via the pipeline.
This can be useful for opening a connection before processing a set of values, then at the end, we can close the connection.
Here is an example of how to generate a list of numbers:
Function Get-Number {
Param([Parameter(ValueFromPipeline=$true)]$Number)
Begin
{
Write-Host "Début de la liste"
}
Process
{
Write-Host ">> $Number"
}
End
{
Write-Host "Fin de la liste"
}
}
Then, we will call this function as follows:
1..15 | Get-Number
This means we will send the numbers 1 to 15 to the function.
On execution, we obtain the following result. We can see that the function has executed the Begin{} and End{} blocks only once. However, each value sent through the pipeline was processed independently.
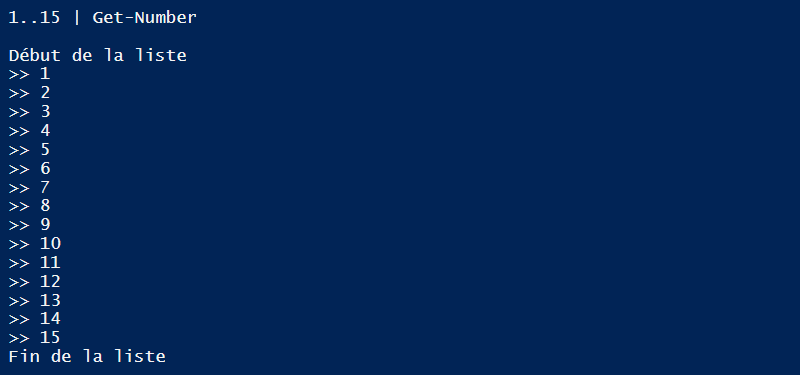
IV. Conclusion
With this simple addition, we've gone from a function to an advanced function in PowerShell. The [CmdletBinding()] instruction is widely used when writing modules so that cmdlets can benefit from these native PowerShell parameters. What's more, using a function structure based on "Begin{}", "Process{}" and "End{}" opens up new possibilities!